Friday, October 06, 2006
Solutions to Chapter 5, p. 92, #3
Question #3 is the only question at the end of the chapter that has a twist or two. It reads:
"Write a program that inputs the starting time and finishing time of an automobile trip as well as the distance in kilometers traveled and outputs the average speed in km/hr. The times are to be given in two parts: the hours, then the minutes."
Begin by trying to deal with the simplest case - for example, a trip that starts at 8 a.m., ends at 10 a.m. and is a distance of 100 km. To begin with you only need two variables - let's call them startHour and endHour - to find how long the trip lasted. So endHour minus startHour gives you the trip in hours. You need another variable called, say, distance. Then to find the average speed in km per hour, divide the hours by the number of km. The Turing code will look like this.
But trips don't always begin and end exactly on the hour. How do we deal with minutes? Convert all the times into minutes, then subtract to give you the time of the trip in minutes. The textbook indicates this method by saying, "the times are to be given in two parts: the hours, then the minutes." Next, you want the result in km per hour. That means distance divided by time (km per hr). But since your time at this point is still in minutes, you divide distance by time then divide the result by 60 to get km per hour. Look carefully at the expression below, especially the brackets.
What if the trip starts in the morning, say 11 a.m., and ends later in the day, say, 4 p.m.? The number 11 is larger than 4. The way to get around this is to ask the user to enter hours using a 24-hour clock:
The final twist to this problem is when the trip lasts beyond midnight, for example from 9 p.m. to 3 a.m. (that is 21 hours to 3 hours). Use an if construct as below. We won't be studying if constructs until Chapter 9, but some of you are already starting to get the idea. Don't worry if this part is confusing. You will learn about it later in the course.
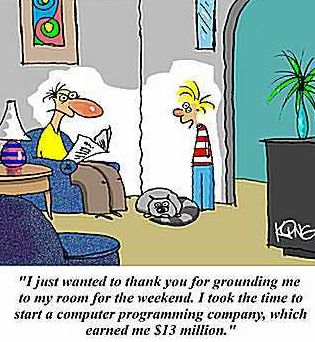
"Write a program that inputs the starting time and finishing time of an automobile trip as well as the distance in kilometers traveled and outputs the average speed in km/hr. The times are to be given in two parts: the hours, then the minutes."
Begin by trying to deal with the simplest case - for example, a trip that starts at 8 a.m., ends at 10 a.m. and is a distance of 100 km. To begin with you only need two variables - let's call them startHour and endHour - to find how long the trip lasted. So endHour minus startHour gives you the trip in hours. You need another variable called, say, distance. Then to find the average speed in km per hour, divide the hours by the number of km. The Turing code will look like this.
var startHour, endHour :int
var distance : real
put "Enter hour when trip started: "
get startHour
put "Enter hour when trip ended: "
get endHour
put "Enter distance of trip: "
get distance
put "Average speed of trip was ", (endHour - startHour) / distance
But trips don't always begin and end exactly on the hour. How do we deal with minutes? Convert all the times into minutes, then subtract to give you the time of the trip in minutes. The textbook indicates this method by saying, "the times are to be given in two parts: the hours, then the minutes." Next, you want the result in km per hour. That means distance divided by time (km per hr). But since your time at this point is still in minutes, you divide distance by time then divide the result by 60 to get km per hour. Look carefully at the expression below, especially the brackets.
var startMin, startHour, endMin, endHour :int
var distance : real
put "Enter hour when trip started: "
get startHour
put "Enter minute when trip started: "
get startMin
put "Enter hour when trip ended: "
get endHour
put "Enter minute when trip ended: "
get endMin
put "Enter distance of trip: "
get distance
put "Average speed of trip was ", distance / (((endHour * 60 + endMin) - (startHour * 60 + startMin)) /60), " km / hr"
What if the trip starts in the morning, say 11 a.m., and ends later in the day, say, 4 p.m.? The number 11 is larger than 4. The way to get around this is to ask the user to enter hours using a 24-hour clock:
put "Enter hour when trip started (use a 24-hour clock): "
get startHour
. . .
put "Enter hour when trip ended (use a 24-hour clock): "
get endHour
. . .
The final twist to this problem is when the trip lasts beyond midnight, for example from 9 p.m. to 3 a.m. (that is 21 hours to 3 hours). Use an if construct as below. We won't be studying if constructs until Chapter 9, but some of you are already starting to get the idea. Don't worry if this part is confusing. You will learn about it later in the course.
. . .
get endHour
if endHour < startHour then
endHour := endHour + 24
end if
. . .
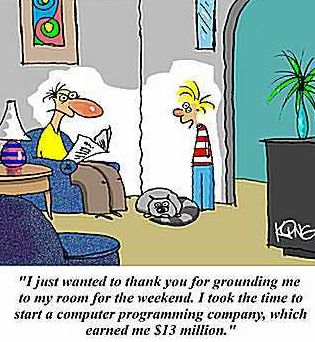
Solutions to Chapter 5, p. 92, #1, 2, 4, 5, 6, 8
Note: These are not the ONLY way of solving the problems. You may have found a way that is different but just as good.
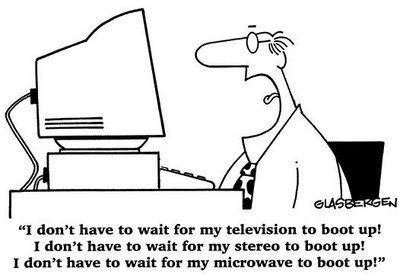
% The "05-01 Solution" program
const factor := 2.54
% number of cm in 1 inch
var len : real
put "Enter the length of the desk in inches: " ..
get len
put "The length of the desk in centimeters is: ", len * factor, " cm."
% The "05-02 Solution" program
const currentYear := 2006
var birthYear : int
put "Enter the year you were born (e.g. 1981):" ..
get birthYear
put "In ", currentYear," you will be ", currentYear - birthYear, " years old"
OR - GET THE USER TO ENTER THE CURRENT YEAR, AS BELOW
var birthYear, thisYear : int
put "Enter the year you were born (e.g. 1981):" ..
get birthYear
put "Enter the current year :"
get thisYear
put "In ", thisYear," you will be ", thisYear - birthYear, " years old."
% The "05-04" Solution" program
var number1, number2, number3, number4 : int
put "Enter 4 numbers"
% Note that all 4 numbers can be entered on one line
get number1, number2, number3, number4
put "Here are the four numbers that you entered: " ..
put number1, ",", number2, ",", number3, ",", number4
% commas in quotation marks, and commas separating items
% The "05-05" Solution" program
const GREETING:= "Hello, "
const PUNCTUATION := "!"
var name : string
put "Enter your name, please"
get name
put GREETING,name,PUNCTUATION
% The "05-06 Solution" program
var number1, number2, number3, product: int
put "Enter 3 numbers"
get number1, number2, number3
product:= number1 * number2 * number3
% the variable product is not necessary but it
% shortens the multiplication expressions below
put "The product of ", number1, " * ", number2, " * ", number3, " = ", answer
put ""
put "The square of ", product, " is ", product**2
% The "Ch05_08" Program
% The two inputs show two methods
var firstName, lastName : string
put "Enter a first name: "
get firstName
% input one string per line
put "Enter a last name: "
get lastName
put lastName, ", ",firstName
put "Enter a first and last name: "
get firstName, lastName
% input two strings per line
put lastName, ", ",firstName
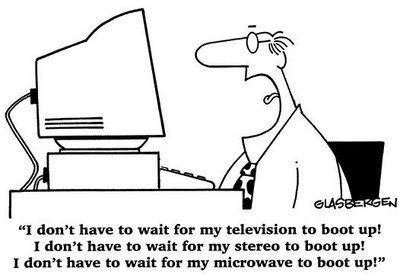